Client Script is the script that runs on the user’s browser. This is the only script that runs on the client-side, all others scripts types execute on the server-side of NetSuite. The primary use of this Script type is for responding to user interactions with records within the NetSuite UI. They can validate user-entered data and auto-populate fields or Sub lists at various form events. Scripts can be run on most standard records, custom record, and custom NetSuite pages like Suitelet.
Functions/trigger events of Client Script
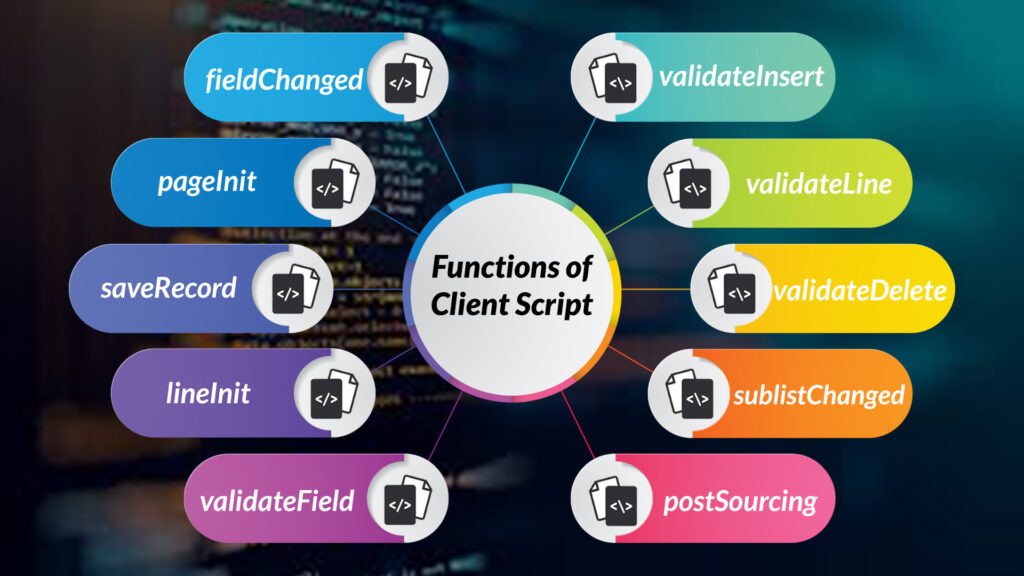
Following triggers can run a client script
- Loading a form for editing
- Adding values or changing field (before and after it is entered)
- Changing a field value that sources another field
- Selecting a line item on a sub list
- Adding a line item (before and after it is entered)
- Saving a form
Client scripts are executed after any existing form-based clients, and before any user event scripts.
Writing your first client script 2.0 code.
define([‘N/ui/dialog’],
function (dialog) {
function showAlertBox(context) {
dialog.alert({
title: ‘I am an Alert’,
message: ‘Hello!’
})
}
return {
pageInit : showAlertBox
});
define() Function will load all the dependencies which the code is executing. For our code, we are using a dialog module. a dialog will provide a pop-up message on the browser screen.
showAlertBox is a pageInit function and will get triggered when we load the page.
dialog.alert will give you one popup when the record loads because we are using pageInit function.
return well let NetSuite understand what triggers we are using in the program.
The above sample shows a client script applied to a sales order form. The script provides a pop-up to the user when the sales order page completes loading.
Client Scripts types with examples:
/**
*@NApiVersion 2.x
*@NScriptType ClientScript
*/
/*
‘NApiVersion 2.x & NScriptType ClientScript’ this will help to netsuite to understand which script and version
we are using where as in 1.0 we have to specify this manually by selection suitescript type and fucntions
used in the script.
*/
define([‘N/error’, ‘N/ui/dialog’, ‘N/log’],
function (error, dialog, log) {
function pageInit(context) { // When the page loads this function will get triggred
if (context.mode !== ‘create’)
return;
dialog.alert({
title: ‘I am an Alert’,
message: ‘PageInit is Working.’
})
}
function validateField(context) { // Validate Field is matching our condition or not, save the field only if it matches our condition
var currentRecord = context.currentRecord;
if (context.fieldId == ‘custbody_phone’) {
var phoneNo = currentRecord.getValue({
fieldId: ‘custbody_phone’
});
var phoneNoCount = phoneNo.length;
if (phoneNoCount < 9) {
dialog.alert({
title: ‘Warning’,
message: ‘Phone No should be 9 digit long.’
})
}
}
return true;
}
function fieldChanged(context) { // check the item id and set value to memo body field //return void
var currentRecord = context.currentRecord;
var sublistNameSo = context.sublistId;
var sublistFieldName = context.fieldId;
var line = context.line;
if (sublistNameSo === ‘item’ && sublistFieldName === ‘item’)
currentRecord.setValue({
fieldId: ‘memo’,
value: ‘Item is : ‘ + currentRecord.getCurrentSublistValue({
sublistId: ‘item’,
fieldId: ‘item’
}) + ‘ is selected’
});
}
function sublistChanged(context) { // this function is triggred when sublist is removed, inserted or edited
var currentRecord = context.currentRecord;
var subNameValueOnSo = context.sublistId;
var op = context.operation;
if (subNameValueOnSo === ‘item’)
currentRecord.setValue({
fieldId: ‘memo’,
value: ‘Sublist item Amount is ‘ + currentRecord.getValue({
fieldId: ‘total’
}) + ‘ and operation is: ‘ + op
});
}
function postSourcing(context) { // the function that is executed when a field that takes information from another field is modified.
var currentRecord = context.currentRecord;
var subNameValueOnSo = context.sublistId;
var sublistFieldName = context.fieldId;
var line = context.line;
if (subNameValueOnSo === ‘item’ && sublistFieldName === ‘item’)
if (currentRecord.getCurrentSublistValue({
sublistId: subNameValueOnSo,
fieldId: sublistFieldName
}) === ‘8235’)
if (currentRecord.getCurrentSublistValue({
sublistId: subNameValueOnSo,
fieldId: ‘amount’
}) < 100)
currentRecord.setCurrentSublistValue({
sublistId: subNameValueOnSo,
fieldId: ‘description’,
value: ‘Amount is less than 100’
});
}
function lineInit(context) { //same as pageInit, triggers when we select sublist//return void
var currentRecord = context.currentRecord;
var subNameValueOnSo = context.sublistId;
if (subNameValueOnSo === ‘partners’)
currentRecord.setValue({
fieldId: ‘custbody_tracking’,
value: currentRecord.getCurrentSublistValue({
sublistId: ‘partners’,
fieldId: ‘partner’
})
});
}
function validateInsert(context) { //selects an existing line in a sublist and then clicks the Insert button
var currentRecord = context.currentRecord;
var subNameValueOnSo = context.sublistId;
if (subNameValueOnSo === ‘partners’)
if (currentRecord.getCurrentSublistValue({
sublistId: subNameValueOnSo,
fieldId: ‘contribution’
}) !== ‘100.0%’)
currentRecord.setCurrentSublistValue({
sublistId: subNameValueOnSo,
fieldId: ‘contribution’,
value: ‘100.0%’
});
return true;
}
function validateDelete(context) { // triggers if we remove sublist
var currentRecord = context.currentRecord;
var subNameValueOnSo = context.sublistId;
if (subNameValueOnSo === ‘partners’)
currentRecord.setValue({
fieldId: ‘custbody_additional_notes’,
value: currentRecord.getCurrentSublistValue({
sublistId: subNameValueOnSo,
fieldId: ‘partner’
}) + ‘ Removed’
});
return true;
}
function validateLine(context) { // Behave like a saveRecord in lineitem, if we save the sublist this function will get triggred
var currentRecord = context.currentRecord;
var subNameValueOnSo = context.sublistId;
if (subNameValueOnSo === ‘partners’)
currentRecord.setValue({
fieldId: ‘custbody_new_partner’,
value: currentRecord.getCurrentSublistValue({
sublistId: subNameValueOnSo,
fieldId: ‘partner’
}) + ‘ Added’
});
return true;
}
function saveRecord(context) { // When we save the record
var options = {
title: “Confirmation”,
message: “Save this record”
};
dialog.confirm(options)
return true;
}
return {
/* this will automatically let the NetSuite understand what function we are using in the code,
pageInit : anyFunctionName (and netsuite will understand this is pageInit Function) */
pageInit: pageInit,
fieldChanged: fieldChanged,
postSourcing: postSourcing,
sublistChanged: sublistChanged,
lineInit: lineInit,
validateInsert: validateInsert,
validateField: validateField,
validateLine: validateLine,
validateDelete: validateDelete,
saveRecord: saveRecord
};
});
- pageInit : This function will get triggered when the page is loaded.
- validateField : This function is executed when a field is changed. The simple example to use this function is by implementing on phone number field.
In the given example code trigger is set on body field ‘Phone No’ if the phone number is less than 9 then give the user a message using pageInit Function that ‘Phone Number should be 9 digit long’. This Field returns true or false
- sublistChanged : This function is executed after a sublist has been inserted, removed, or edited. ex: If you insert item on sales order. In the above example if you add, remove or edit the operation well get displayed on memo field.
- postSourcing : This function is executed when a field that takes value from another field is modified. ex: If you have 2 field then one field can be modified by values of another field.
- lineInit : This is same as pageInit i.e it gets triggers when we click on line items. In the above example when you click on partner it will give you the internal id.
- validateInsert : This validation function is executed when a sublist line is inserted into an edit sublist. returns true/false i.e if returns false then don’t save sublist.
- validateDelete : When the sublist line is removed this type will get triggered.
- validateLine : When we save the record this function will get triggered.
- fieldChanged : This field will get triggered when a field is changed by the user. the fieldChange type returns void.
- saveRecord : This function well get executed when we save the record.
For reference, comments are added in the scripts |
Follow the steps to use the above code:
- Copy the above code and save with .js extension.
- Navigate to – customization > Scripting > Scripts >new & upload the file.
- Deploy the script on – sales order. (You can deploy client script on any record).
- Now you can create a new sales order and practice.
More About Client Script:
- Client Script cannot be debugged in NetSuite debugger.
- Client script executes only on created, copied and edited records. If you want to run in other mode use user event Script.
- The governance limit of client script is 1000 units.
Learn More: Client Script & Client Script 2.0
To Know more about NetSuite Cloud ERP, feel free to reach us on Website: https://saturotech.com/
Email ID: sales@saturotech.com
Contact No: +91 844-844-8939 (& Press 3)